Use the event syntax (eventName) to make your application respond to user input.
You can specify the event handler—a method in the component controller—like this:
As in previous examples, you can make element references available to
other parts of the template as a local
variable using the # syntax.
Using #
and events,
you can write the old "update text as you type" example:
In that example, #myname
creates a local variable in the template that
the <p>
element can refer to.
The (keyup)
tells Angular to trigger updates when it gets a keyup
event. And {{myname.value}}
binds the text node of the
<p>
element to the
input's value property.
Let's do something a little more complex, where the user enters items that the app adds to a list:
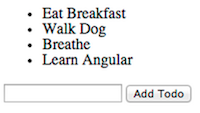
Create a list property
With the default files in place, create a TodoController class to manage interactions with the list. Inside TodoController, add a list with some initial items. Then add a method that adds new items to the list.
As shown in the previous example, a production application you would
separate the model out into another class
and inject it into TodoController
.
We've omitted that here for brevity.
Display the list of todos
Using the *ng-for
iterator, create an <li>
for each item in the todos list and set
its text to the value.
Add todos to the list via button click
Now, add a text input and a button for users to add items to the list. As you saw above, you can create a local
variable reference in your template with #varname
. Call it #todotext
here.
Specify the target of the click event binding as your controller's
addTodo()
method and pass
it the value. Since you created a reference called todotext
,
you can get the value with todotext.value.
To make pressing Enter do something useful, you can add a keyup event handler to the input field. This event handler uses APIs defined in dart:html, so be sure to import that library.