Let's walk through how to display a property and a list of properties, and then to conditionally show content based on state. The final UI looks like this:
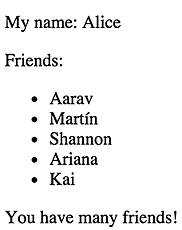
Create entry points and pubspec
Open your favorite editor and create a directory with
a web/main.dart
file,
a web/index.html
file, and
a pubspec.yaml
file:
All of this code should look familiar from the previous page,
except for the imports in main.dart
.
The import of show_properties.dart
lets you implement part of the app in a different Dart file.
You've previously seen angular2.dart
imported,
but that's unnecessary here because this version of main.dart
doesn't implement any components or other injectable types.
All three of these files remain similar in the rest of the examples, so we'll focus on what changes.
Showing properties with interpolation
The simple method for binding text into templates is through interpolation, where you put the name of a property inside {{ }}.
To see this working, first create a lib
directory.
Under it, put a Dart file named show_properties.dart
that contains the following code:
My name: {{ myName }}
''') class DisplayComponent { String myName = 'Alice'; }You've just defined a component that encompasses a view and controller for the app. The view defines a template:
My name: {{ myName }}
Angular will automatically pull the value of myName
and
insert it into the browser,
automatically updating it whenever it changes.
While you've used template:
to specify an inline view, for larger templates you'd
want to move them to a separate file and load them with templateUrl:
instead.
One thing to notice is that although you've written
your DisplayComponent
class, you haven't
used new
to instantiate it.
Because your class is associated with <display>
elements in
the DOM, Angular automatically calls new
on
DisplayComponent
and bind its properties to
that part of the template.
When you're building templates, data bindings like these have access to
the same scope of
properties as your controller class does.
Here your class is DisplayComponent
, which has
just one property, myName
.
Add a second line to the template, so you can see Angular dynamically update content:
Then import dart:async
so you can use a Timer,
and give the DisplayComponent
a starting value for time and
create a periodic Timer call to update the time:
Reload the app, and you'll now see the seconds updating automatically.
Display an iterable using *ng-for
Moving up from a single value, create a property that's a list of values.
You can then use this list in your template with
the ng-for
directive to create copies of DOM elements
with one for each item in the list.
To make ng-for
work,
you need to add the Angular NgFor
directive,
so that Angular knows to include it.
Add NgFor
using the optional directives
parameter.
Reload and you've got your list of friends!
Again, Angular will mirror changes you make to this list over in the DOM. Add a new item and it appears in your list. Delete one and Angular deletes the <li>. Reorder items and Angular makes the corresponding reorder of the DOM list.
Let's look at the few lines that do the work again:
The way to read this is:
*ng-for
: Create a DOM element for each item in an iterable such as a list.#name
: Refer to individual values of the iterable asname
.of friendNames
: The iterable to use is calledfriendNames
in the current controller.
Using this syntax, you can build UI lists from any iterable object.
Create a model and inject it
Before we get too much further, we should mention that putting the model (list) directly into the controller isn't proper form. We should separate the concerns by having another class serve the role of model and inject it into the controller.
Make a FriendsService
class to implement a model
containing a list of friends.
Put this in a new file under lib/
named friends_service.dart
. Here's what the class looks like:
Now you can replace the current list of friends in DisplayComponent.
Import friends_service.dart
,
and add a FriendsService parameter to the constructor.
Then set friendNames
to the names provided by the service.
Next, make FriendsService available to dependency injection
by adding a viewProviders
parameter to DisplayComponent's
@Component
annotation:
Conditionally display data using *ng-if
Lastly, before we move on, let's handle showing parts of our UI conditionally with *ng-if
. The
NgIf
directive adds or removes elements from the DOM based on the expression you provide.
See it in action by adding a paragraph at the end of your template:
Also add NgIf
to the list of directives,
so Angular knows to include it:
The list currently has 5 items, so if you run the app you'll see the message congratulating you on your many friends. Remove two items from the list, reload your browser, and see that the message no longer displays.
Here's the final code.
Explanations
Using multiple Dart files in an Angular app
Dart offers a few ways to implement an app in multiple files. In this guide, each example is in a single package, and each Dart file implements a separate library. For a bigger project, you might split the code into libraries in two or more packages.
To use the API defined in show_properties.dart
,
main.dart
must import that file.
The import statement uses the package name
(defined in pubspec.yaml
to be displaying_data)
and the path to show_properties.dart
(starting at the app's top directory,
but omitting the lib/
directory).
The name that show_properties.dart
specifies for its library
is similar to the path used to import the library,
but with no ".dart" suffix and
with dots (.
) instead of slashes (/
).
Naming
conventions for libraries,
along with lots of other helpful information, are in the
Dart Style Guide.
Another import lets show_properties.dart
use the API defined in friends_service.dart
:
Because both show_properties.dart
and friends_service.dart
are under lib
,
the import could instead use a relative path:
The app's entry point—main.dart
—imports
bootstrap.dart
so that it can call bootstrap()
.
Both show_properties.dart
and friends_service.dart
import angular2.dart
so that they can define Angular components and models.
For more information on implementing Dart libraries, see Libraries and visibility in the Dart language tour.