We’ve all used a form to log in, submit a help request, place an order, book a flight, schedule a meeting, and perform countless other data entry tasks. Forms are the mainstay of business applications.
Any seasoned web developer can slap together an HTML form with all the right tags. It's more challenging to create a cohesive data entry experience that guides the user efficiently and effectively through the workflow behind the form.
That takes design skills that are, to be frank, well out of scope for this chapter.
It also takes framework support for two-way data binding, change tracking, validation, and error handling ... which we shall cover in this chapter on Angular forms.
We will build a simple form from scratch, one step at a time. Along the way we'll learn:
How to build an Angular form with a component and template
The
ngModel
two-way data binding syntax for reading and writing values to input controlsThe
ngControl
directive to track the change state and validity of form controlsThe special CSS classes that
ngControl
adds to form controls and how to use them to provide strong visual feedbackHow to display validation errors to users and enable/disable form controls
How to share information across controls with template local variables
Template-driven forms
Many of us will build forms by writing templates in the Angular template syntax with the form-specific directives and techniques described in this chapter.
That's not the only way to create a form but it's the way we'll cover in this chapter.
We can build almost any form we need with an Angular template—login forms, contact forms, pretty much any business form. We can lay out the controls creatively, bind them to data, specify validation rules and display validation errors, conditionally enable or disable specific controls, trigger built-in visual feedback, and much more.
It will be pretty easy because Angular handles many of the repetitive, boilerplate tasks we'd otherwise wrestle with ourselves.
We'll discuss and learn to build a template-driven form that looks like this:
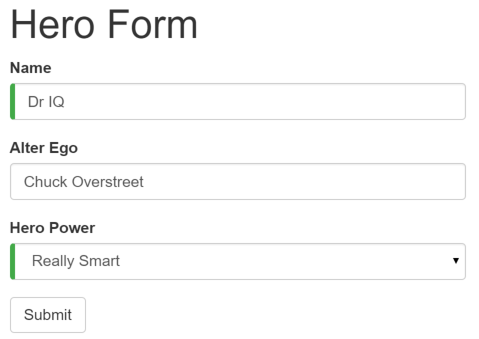
Here at the Hero Employment Agency we use this form to maintain personal information about the heroes in our stable. Every hero needs a job. It's our company mission to match the right hero with the right crisis!
Two of the three fields on this form are required. Required fields have a green bar on the left to make them easy to spot.
If we delete the hero name, the form displays a validation error in an attention-grabbing style:
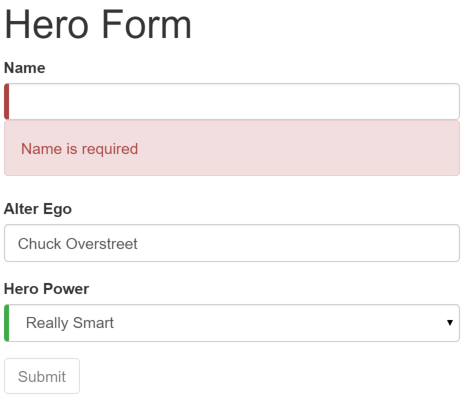
Note that the submit button is disabled, and the "required" bar to the left of the input control changed from green to red.
We'll customize the colors and location of the "required" bar with standard CSS.
We'll build this form in small steps:
- Create the
Hero
model class. - Create the component that controls the form.
- Create a template with the initial form layout.
- Add the ngModel directive to each form input control.
- Add the ngControl directive to each form input control.
- Add custom CSS to provide visual feedback.
- Show and hide validation error messages.
- Handle form submission with ngSubmit.
- Change the form's display after submission.
Setup
Create a new project folder (angular2_forms
) and create 3 files:
pubspec.yaml
, web/index.html
, and web/main.dart
.
(These files should be familiar from the
QuickStart.) Put these contents in the files:
So that the code can run,
let's create a stub for the <hero-form>
component.
Create a new directory called lib
.
In it, put a file called hero_form_component.dart
with the following code:
The app should now run, but it won't do anything interesting. Let's add some data.
Create the Hero model class
As users enter form data, we'll capture their changes and update an instance of a model. We can't lay out the form until we know what the model looks like.
A model can be as simple as a "property bag" that holds facts about a thing of application importance.
That describes well our Hero
class with its three required fields (id
, name
, power
)
and one optional field (alterEgo
).
In the lib
directory, add a file called hero.dart
with the following code:
It's an anemic model with few requirements and no behavior. Perfect for our demo.
The alterEgo
is optional, so the constructor lets us omit it: note the
[]
in [this.alterEgo]
.
We can create a new hero like this:
Create a form component
An Angular form has two parts: an HTML-based template and a code-based component to handle data and user interactions.
We begin with the component because it states, in brief, what the Hero editor can do.
Edit hero_form_component.dart
, replacing all of its contents
with the following code:
There’s nothing special about this component, nothing to distinguish it from any component we've written before,
nothing form-specific about it ... except, perhaps, the tell-tale FORM_DIRECTIVES
import.
[PENDING: Soon that import shouldn't be necessary.]
Understanding this component requires only the Angular 2 concepts covered in previous chapters.
The code imports a standard set of symbols from the Angular library.
The
@Component
selector value of "hero-form" means we can drop this form in a parent template with a<hero-form>
tag.The
templateUrl
property points to a separate file for template HTML calledhero_form_component.html
.We defined dummy data for
model
andpowers
, as befits a demo. Down the road, we can inject a data service to get and save real data or perhaps expose these properties as inputs and outputs for binding to a parent component. None of this concerns us now, and these future changes won't affect our form.We threw in a
diagnostic
property to return a string describing our model. It'll help us see what we're doing during our development; we've left ourselves a cleanup note to discard it later.
Why isn't the template inline in the component file?
Inline templates can be nice when they are short, but most form templates aren't short. Dart files generally aren't the best place to write (or read) large stretches of HTML, and few editors are much help with files that have a mix of HTML and code. It's also nice to have short files with a clear and obvious purpose.
We made a good choice to put the HTML template elsewhere. Let's write it.
Create an initial HTML form template
Create a new file under lib
called hero_form_component.html
,
and put the following template code in it:
That is plain old HTML 5. We're presenting two of the Hero
fields, name
and alterEgo
, and
opening them up for user input in input boxes.
The Name <input>
control has the HTML5 required
attribute;
the Alter Ego <input>
control does not because alterEgo
is optional.
We've got a Submit button at the bottom with some classes on it.
We are not using Angular yet. There are no bindings. No extra directives. Just layout.
The container
,form-group
, form-control
, and btn
classes are Bootstrap CSS. Purely cosmetic.
We're using Bootstrap to gussy up our form.
Hey, what's a form without a little style!
Angular makes no use of the container
, form-group
, form-control
, and btn
classes or
the styles of any external library. Angular apps can use any CSS library
... or none at all.
Let's add the stylesheet.
Download the Bootstrap stylesheet from https://maxcdn.bootstrapcdn.com/bootstrap/3.3.5/css/bootstrap.min.css, and put it in the
web
directory.Edit
web/index.html
, adding a link tobootstrap.min.css
:
Add powers with *ngFor
Our hero must choose one super power from a fixed list of Agency-approved powers.
We maintain that list internally (in HeroFormComponent
).
We'll add a select
to our
form and bind the options to the powers
list using NgFor
,
a technique used before in Displaying Data.
Add the following HTML immediately below the Alter Ego group.
This code repeats the <option>
tag for each power in the list of powers.
The #p
local template variable is a different power in each iteration;
we display its name using the interpolation syntax with the double curly braces.
Two-way data binding with *ngModel
Running the app right now would be disappointing.
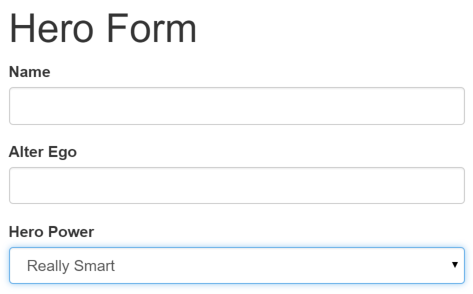
We don't see hero data because we are not binding to the Hero
yet.
We know how to do that from earlier chapters.
Displaying Data taught us property binding.
User Input showed us how to listen for DOM events with an
event binding and how to update a component property with the displayed value.
Now we need to display, listen, and extract at the same time.
We could use the techniques we already know, but
instead we'll introduce something new: the NgModel
directive, which
makes binding the form to the model super easy.
Find the <input>
tag for Name and update it like this:
We added a diagnostic interpolation after the input tag so we can see what we're doing. We left ourselves a note to throw it way when we're done.
Focus on the binding syntax: [(ngModel)]="..."
.
If we ran the app right now and started typing in the Name input box, adding and deleting characters, we'd see them appearing and disappearing from the interpolated text. At some point it might look like this.

The diagnostic is evidence that values really are flowing from the input box to the model and back again. That's two-way data binding!
Let's add similar [(ngModel)]
bindings to Alter Ego and Hero Power.
We'll ditch the input box binding message
and add a new binding at the top to the component's diagnostic
property.
Then we can confirm that two-way data binding works for the entire Hero model.
After revision, the core of the form should have three [(ngModel)]
bindings that
look much like this:
If we ran the app right now and changed every Hero model property, the form might look like this:
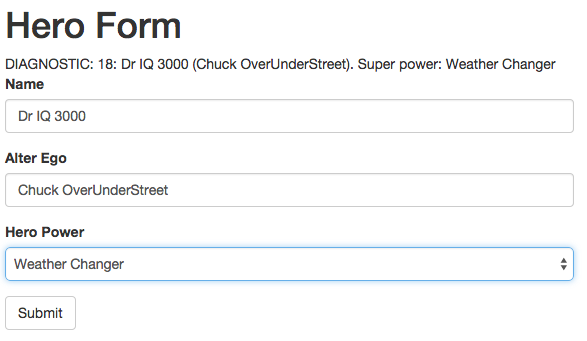
The diagnostic near the top of the form confirms that our changes to the values are reflected in the model.
We're done with the diagnostic binding. Delete it now.
Inside [(ngModel)]
This section is an optional deep dive into [(ngModel)]. Not interested? Skip ahead!
The punctuation in the binding syntax, [()], is a good clue to what's going on.
A property binding makes data flow from the model to a target property on screen. We identify that target property by surrounding its name in brackets, []. This is a one-way data binding from the model to the view.
An event binding makes data flow from the target property onscreen to the model. We identify that target property by surrounding its name in parentheses, (). This is a one-way data binding in the opposite direction from the view to the model.
No wonder Angular uses the combined punctuation, [()], to signify a two-way data binding and a flow of data in both directions.
In fact, we can break the NgModel
binding into its two separate modes
as in this rewrite of the Name <input>
binding:
The property binding should feel familiar. The event binding might seem strange.
The name ngModel-change
specifies an event property of the NgModel
directive.
When Angular sees a binding target in the form [(abc)],
it expects the abc
directive to have an abc
input property and an abc-change
output property.
The other oddity is the template expression, model.name = $event
.
We're used to seeing an $event
object coming from a DOM event.
The ngModel-change
property doesn't produce a DOM event; it's an Angular EventEmitter
property that returns the input box value when it fires—which is precisely what
we should assign to the model's name
property.
Nice to know but is it practical? [(ngModel)]
is usually what we want, but
we might split the binding when the event handling has to do something special
such as debounce or throttle the keystrokes.
Track change-state and validity with ngControl
A form isn't just about data binding. We'd also like to know the state of the controls on our form.
The NgControl
directive keeps track of control state for us.
The NgControl
is one of a family of NgForm
directives that can only be applied to
a control within a <form
> tag.
Our application can ask an NgControl
instance whether
the user touched the control, the value changed, or the value is valid.
NgControl
doesn't just track state; it updates the control with special
Angular CSS classes, such as ng-valid
or ng-invalid
.
We can use those class names to change the appearance of the
control and make messages appear or disappear.
We'll explore those effects soon. Right now
let's add ngControl
to all three form controls,
starting with the Name input box.
Be sure to assign a unique name to each ngControl
directive.
Angular registers controls under their ngControl
names
with the NgForm
directive.
We didn't add the NgForm
directive explicitly but it's here;
we'll talk about it later in this chapter.
Add custom CSS for visual feedback
NgControl
doesn't just track state. It updates the control to
have three classes that describe the control's state.
State | Class if true | Class if false |
---|---|---|
Control has been visited | ng-touched | ng-untouched |
Control's value has changed | ng-dirty | ng-pristine |
Control's value is valid | ng-valid | ng-invalid |
Let's add a temporary local template variable
named spy
to the Name <input>
tag and use the spy to display those classes.
Now run the app, and look at the Name input box. Follow the next four steps precisely:
- Look but don't touch.
- Click inside the name box, then click outside it.
- Add slashes to the end of the name.
- Erase the name.
The classes are displayed as follows:
form-control ng-untouched ng-valid ng-pristine
(initial state)form-control ng-valid ng-pristine ng-touched
(after clicking)form-control ng-valid ng-touched ng-dirty
(after changing)form-control ng-touched ng-dirty ng-invalid
(after erasing)

The (ng-valid
| ng-invalid
) pair are the most interesting to us, because we want to send a
strong visual signal when the values are bad. We also want to mark required fields.
We can do both at the same time with a colored bar on the left of the input box:
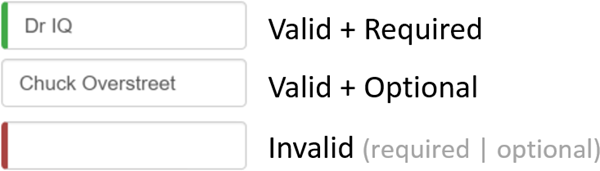
We achieve this effect by adding two styles to a new styles.css
file
(under web/
).
These styles select for the two Angular validity classes and the HTML 5 "required" attribute.
To add the styles to the app,
update the <head>
of index.html
to link to styles.css
.
Show and hide validation error messages
We can do better.
The Name input box is required. Clearing it turns the bar red. That says something is wrong but we
don't know what is wrong or what to do about it.
We can leverage the ng-invalid
class to reveal a helpful message.
Here's the way it should look when the user deletes the name:
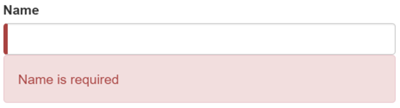
To achieve this effect we need to add two chunks of code:
- A local template variable in the
<input>
tag. - The "is required" message in a nearby
<div>
. We'll display this message only if the control is invalid.
Here's an example of adding an error message to the "name" input box:
We initialized the template local variable with the string "ngForm"
(#name="ngForm"
).
Angular recognizes that syntax and sets the name
variable
to the Control
object identified by the ngControl
directive that,
not coincidentally, we called "name".
We bind the Control
object's valid
property to the element's hidden
property.
While the control is valid, the message is hidden;
if it becomes invalid, the message is revealed.
The NgForm directive
Recall from the previous section that ngControl
registered this input box with the
NgForm
directive as "name".
We didn't add the NgForm
directive explicitly.
Angular added it surreptitiously, wrapping it around the <form>
element.
The NgForm
directive supplements the <form>
element with additional features.
It collects controls (elements identified by an ngControl
directive)
and monitors their properties including their validity.
It has its own valid
property, which is true only if every contained
control is valid.
In this example, we are pulling the "name" control out of its controls
collection
and assigning it to a template local variable so that we can
access the control's properties—such as the control's own valid
property.
The Alter Ego is optional so we can leave that be.
Hero Power selection is required.
We can add the same kind of error handling to the <select>
if we want,
but it's not imperative because the selection box already constrains the
power to valid values.
Submit the form with ngSubmit
The user should be able to submit this form after filling it in.
The Submit button at the bottom of the form
does nothing on its own, but it will
trigger a form submit because of its type (type="submit"
).
A "form submit" is meaningless at the moment. To make it meaningful,
we'll update the <form>
tag with another Angular directive, NgSubmit
,
and bind it to the HeroFormComponent.onSubmit()
method:
We slipped in something extra there at the end! We defined a
template local variable, #heroForm
, and initialized it with the value "ngForm".
The variable heroForm
is now a handle to the NgForm
as we discussed earlier
with respect to ngControl
, although this time we have a reference to the form
rather than a control.
We'll bind the form's overall validity via
the heroForm
variable to the button's disabled
property
using an event binding. Here's the code:
If we run the application now, we find that the button is enabled. It doesn't do anything useful yet but it's alive.
Now if we delete the Name, we violate the "required" rule, which is duly noted in the error message. The Submit button is also disabled.
Not impressed? Think about it for a moment. What would we have to do to wire the button's enable/disabled state to the form's validity without Angular's help?
For us, it was as simple as:
- Define a template local variable on the (enhanced) form element.
- Refer to that variable in a button many lines away.
Toggle two form regions (extra credit)
Submitting the form isn't terribly dramatic at the moment.
An unsurprising observation for a demo. To be honest, jazzing it up won't teach us anything new about forms. But this is an opportunity to exercise some of our newly won binding skills. If you aren't interested, go ahead and skip to this chapter's conclusion.
Let's do something more strikingly visual. Let's hide the data entry area and display something else.
Start by wrapping the form in a <div>
and binding
its hidden
property to the HeroFormComponent.submitted
property.
The main form is visible from the start because the
submitted
property is false until we submit the form,
as the following code from hero_form_component.dart
shows:
When we click the Submit button, the submitted
flag becomes true and the form disappears
as planned.
Now the app needs to show something else while the form is in the submitted state.
Add the following block of HTML below the <div>
wrapper we just wrote:
There's our hero again, displayed read-only with interpolation bindings. This slug of HTML appears only while the component is in the submitted state.
The HTML includes an Edit button whose click event is bound to an expression
that clears the submitted
flag.
When we click the Edit button, this block disappears and the editable form reappears.
That's as much drama as we can muster for now.
Conclusion
The Angular 2 form discussed in this chapter takes advantage of the following framework features to provide support for data modification, validation, and more:
- An Angular HTML form template.
- A form component class with a
Component
decorator. - The
ngSubmit
directive for handling the form submission. - Template local variables such as
#heroForm
,#name
,#p
, and#spy
. - The
ngModel
directive for two-way data binding. - The
ngControl
directive for validation and form element change tracking. - The local variable’s
valid
property on input controls to check if a control is valid and show/hide error messages. - Property binding to disable the submit button when the form is invalid.
- Custom CSS classes that provide visual feedback to users about required invalid controls.
Here’s the code for the final version of the application: