Responding to user input with event syntax
You can make your application respond to user input by using the event syntax. The event syntax starts with an event name surrounded by parenthesis: (event)
. A controller function is then assigned to the event name: (event)="controllerFn()"
.
For a particular control like an input you can have it call methods on your controller on keyup event like so:
Local variables
As in previous examples, you can make element references available to other parts of the template as a local
variable using the #var
syntax. With this and events, we can do the old "update text as you type" example:
The #myname
creates a local variable in the template that we'll refer to below in the
<p>
element. The (keyup)
tells Angular to trigger updates when it gets a keyup
event. And the {{myname.value}}
binds the text node of the <p>
element to the
input's value property.
Let's do something a little more complex where users enter items and add them to a list like this:
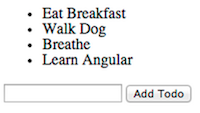
Create an array property
With the default bootstrapping in place, create a controller class that will manage interactions with the list. Inside the controller, add an array with an initial list of items. Then add a method that pushes new items on the array when called.
As with the previous example, in a production application you will separate your model out into another class
and inject it into TodoList
. We've omitted it here for brevity.
Display the list of todos
Using the *ng-for
iterator, create an <li>
for each item in the todos array and set
its text to the value.
Add todos to the list via button click
Now, add a text input and a button for users to add items to the list. As you saw above, you can create a local
variable reference in your template with #varname
. Call it #todotext
here.
Lastly, specify the target of the click event binding as your controller's addTodo()
method and pass
it the value. Since you created a reference called todotext
, you can get the value with
todotext.value.
And then create the doneTyping()
method on TodoList and handle adding the todo text.